Generic Filter (Majority)¶
With the Spatial Generic Filter Processing Algorithm you can use the scipy generic_filter function to write your own spatial filter functions.
In this example we will write a mode filter (also known as majority filter). This kind of filter is often used to smooth classification results (reduce salt-and-pepper effects in the image). It calculates the mode of all values in a specifiable neighborhood around a pixel (e.g. 3x3 pixel window) and assigns this value to the pixel.
In the Processing Toolbox go to and open
Select an input raster under Raster
In the Code text window you can enter python code. Delete the existing content and enter the following
from scipy.ndimage.filters import generic_filter import numpy as np from scipy.stats import mode def filter_function(invalues): invalues_mode = mode(invalues, axis=None, nan_policy='omit') return invalues_mode[0] function = lambda array: generic_filter(array, function=filter_function, size=3)
Specify the output raster location and click Run
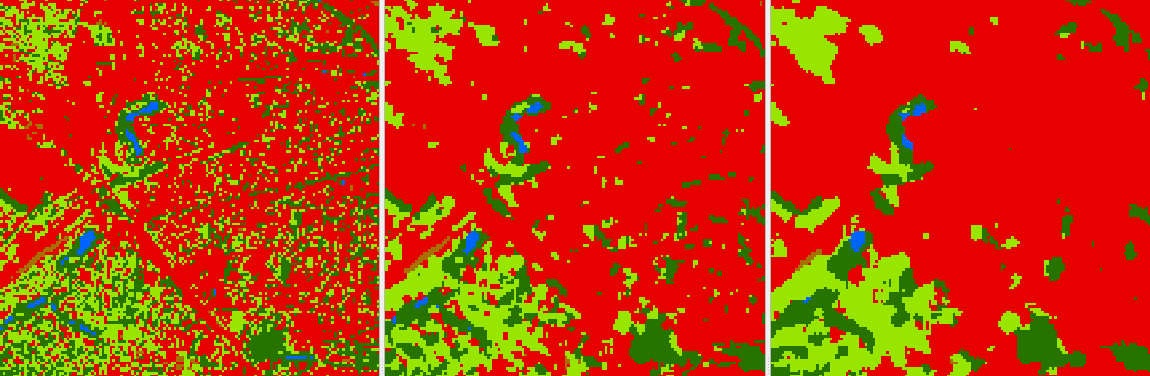
Majority filter applied to classification image: original classification (left), majority filtered 3x3 window (middle), majority filtered 5x5 window (right)
Tip
Also have a look at the scipy.stats.mode documentation. You can change
the window size by altering the size
parameter in the generic_filter
function.
You could further improve the function above by putting constraints on the definition of majority (for example, only update the original value if the frequency of the modal value is higher than 50 percent)